EventBus介绍
EventBus 是一种事件发布订阅模式(观察者模式),通过 EventBus 我们可以很方便的实现解耦,将事件的发起和事件的处理的很好的分隔开来,很好的实现解耦。 微软官方的示例项目 EShopOnContainers 也有在使用 EventBus 。
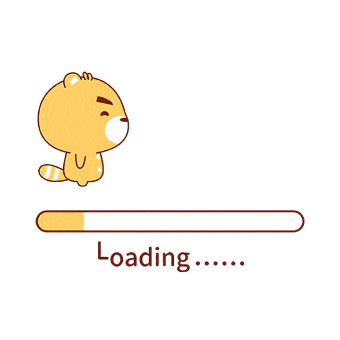
这里的 EventBus 实现也是参考借鉴了微软 eShopOnContainers 项目。
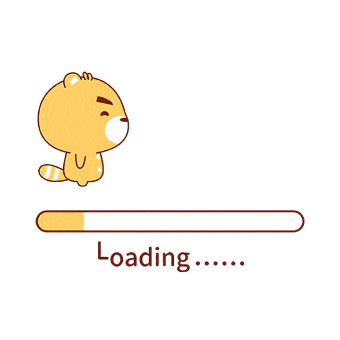
EventBus整体架构
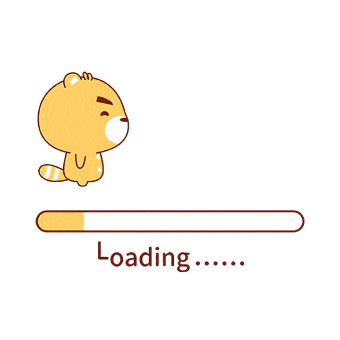
IEventBase
: 事件消息定义接口,所有事件消息都继承这个接口,这个接口定义了事件消息唯一Id EventId
和事件消息发生时间 EventAt
。
IEventBase
1 2 3 4 5 6
| public interface IEventBase { string EventId { get; }
DateTimeOffset EventAt { get; } }
|
IEventHandler
: 定义了一个 Handle
方法来处理相应的事件
IEventHandler
1 2 3
| public interface IEventHandler { }
|
IEventHandler<T>
1 2 3 4 5
| public interface IEventHandler<in TIEvent> : IEventHandler where TIEvent : IEventBase { Task Handle(TIEvent @event); }
|
IDynamicEventHandler
1 2 3 4
| public interface IDynamicEventHandler:IEventHandler { Task Handle(dynamic @event); }
|
IEventStore
: 所有的事件的处理存储,保存事件的IEventHandler,一般不会直接操作,通过 EventBus 的订阅和取消订阅来操作 EventStore
IEventStore
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| public interface IEventStore {
event EventHandler<string> OnEventRemoved;
bool IsEmpty();
void AddSubscription<TEvent, TEventHandler>() where TEventHandler : IEventHandler<TEvent> where TEvent : IEventBase;
void AddDynamicSubscription<TEventHandler>(string eventName) where TEventHandler : IDynamicEventHandler;
void RemoveSubscription<TEvent, TEventHandler>() where TEventHandler : IEventHandler<TEvent> where TEvent : IEventBase;
void RemoveDynamicSubscription<TEventHandler>(string eventName) where TEventHandler : IDynamicEventHandler;
bool HasSubscriptionsForEvent<TEvent>() where TEvent : IEventBase;
bool HasSubscriptionsForEvent(string eventName);
Type GetEventTypeByName(string eventName);
IEnumerable<SubscriptionInfo> GetHandlersForEvent<TEvent>() where TEvent : IEventBase;
IEnumerable<SubscriptionInfo> GetHandlersForEvent(string eventName);
void Clear();
string GetEventKey<T>(); }
|
IEventBus
: 用来发布/订阅/取消订阅事件,并将事件的某一个 IEventHandler 保存到 EventStore 或从 EventStore 中移除
注意:这里我把接口再拆分成 IPublisher
和 ISubscriber
便于理解
IEventBus
1 2 3
| public interface IEventBus : IPublisher, ISubscriber { }
|
IPublisher
1 2 3 4
| public interface IPublisher { void Publish<TEvent>(TEvent @event) where TEvent : IEventBase; }
|
ISubscriber
1 2 3 4 5 6 7 8 9 10
| public interface ISubscriber { void Subscribe<TEvent, TEventHandler>() where TEventHandler : IEventHandler<TEvent> where TEvent : IEventBase;
void SubscribeDynamic<TEventHandler>(string eventName) where TEventHandler : IDynamicEventHandler;
void Unsubscribe<TEvent, TEventHandler>() where TEventHandler : IEventHandler<TEvent> where TEvent : IEventBase;
void UnsubscribeDynamic<TEventHandler>(string eventName) where TEventHandler : IDynamicEventHandler; }
|
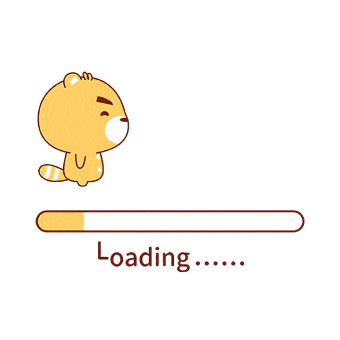
EventBus实现
具体实现代码就不贴了,github上很多,类库也有很多,这里只是记录下整体结构,干了件什么事情。
总结
以前只管使用,不关心具体怎么做,感觉写代码就像虚的,而不是那种实实在在的感觉。
其实具体做了什么很简单。没有那么多深奥的东西在,整理了一下学习的过程。